Getting started with Terraform
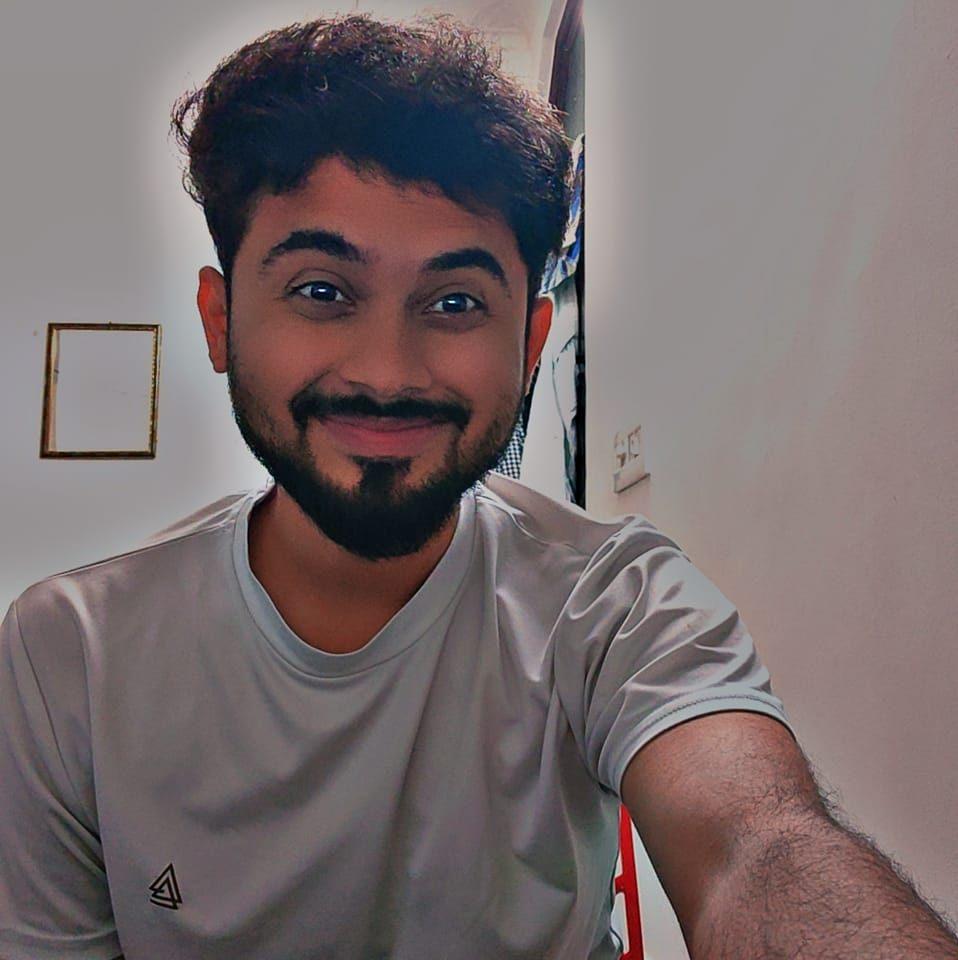
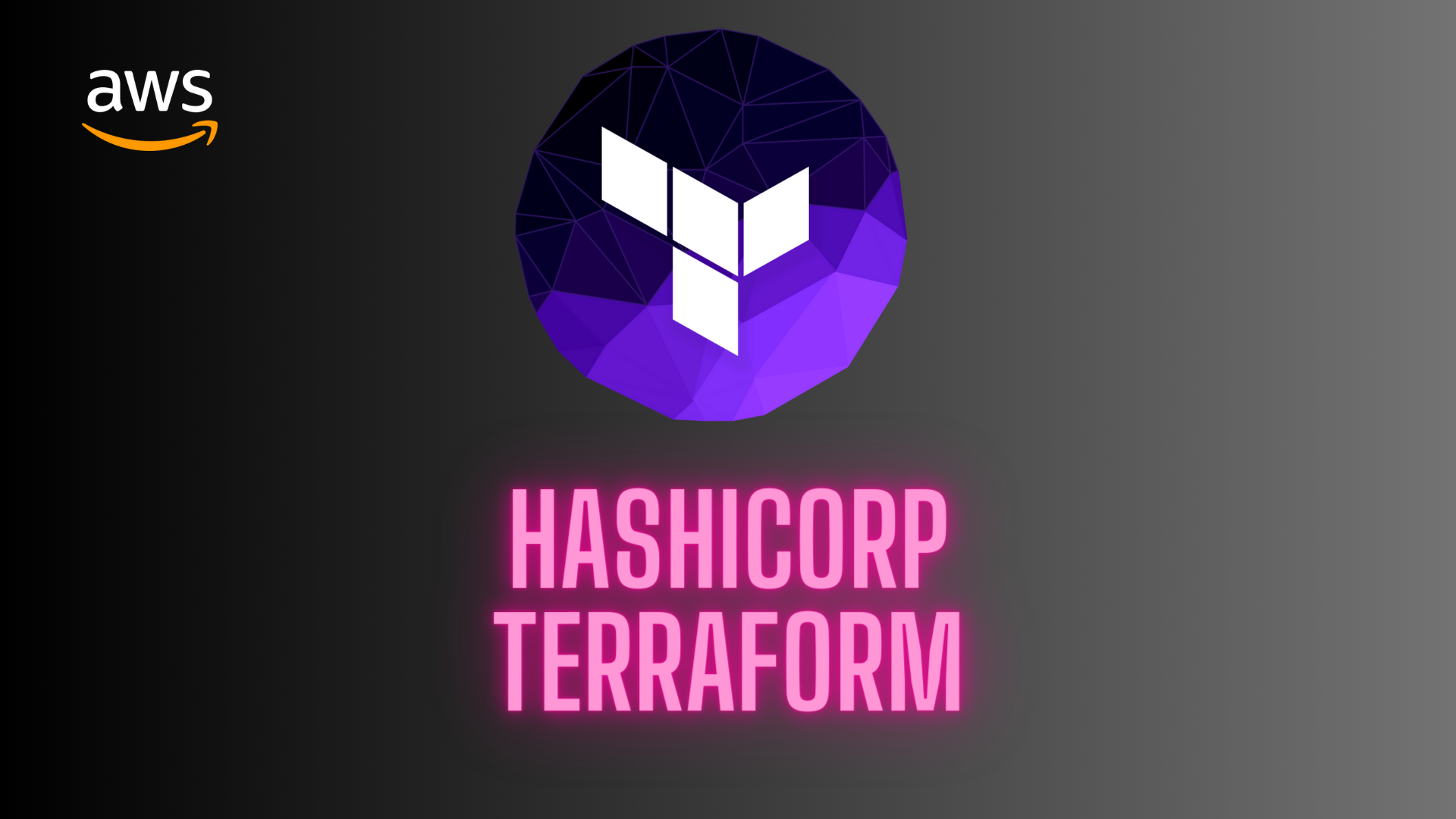
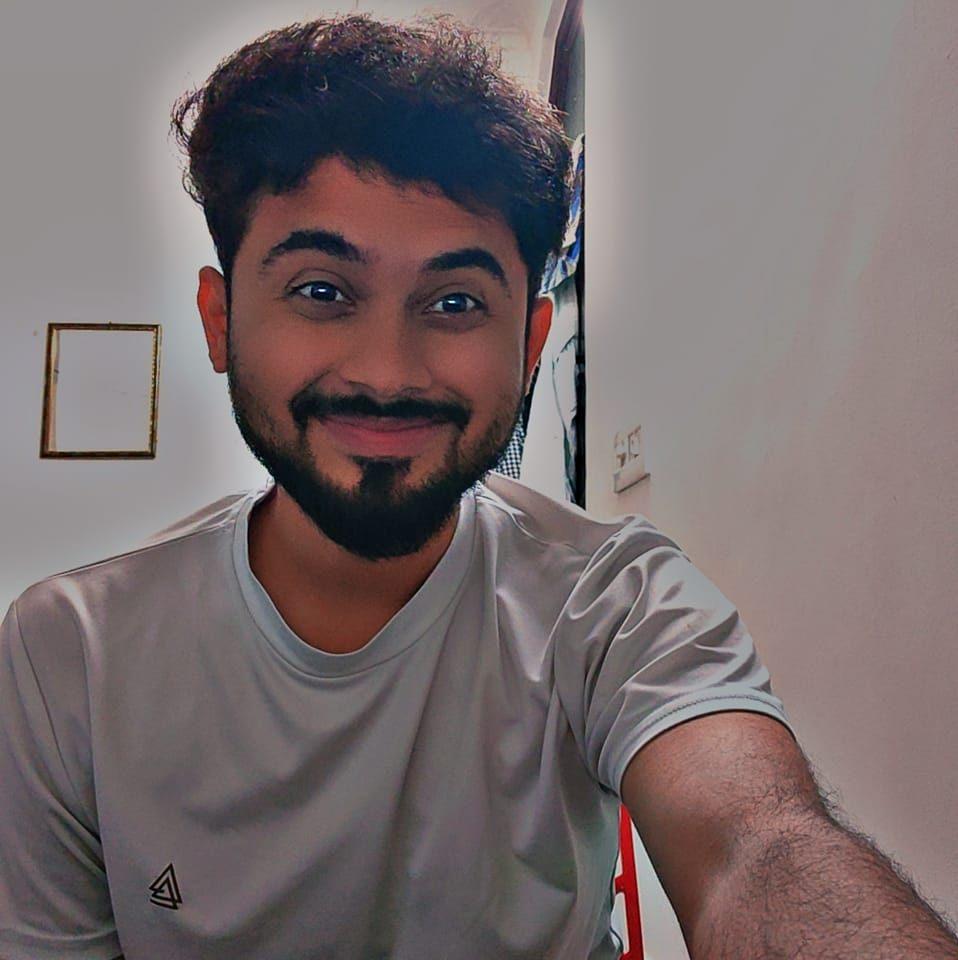
What is Terraform
Terraform is a Infrastructure provisioning tool. Terraform follows IaC (Infrastructure as Code) approach. Terraform can maintain the infrastructure with the help of code and keep track of the state of infrastructure.
Terraform AWS Provider
Terraform support multiple provider like Azure, Google, Kubernetes. We will look how to start with Terraform AWS provider.
For Terraform AWS provider, first we have to export ACCESS_KEY_ID and SECRET_ACCESS_KEY variable in our teminal. For Windows use git bash. Generate these keys in your AWS account and export like this in your terminal.
export AWS_ACCESS_KEY_ID=<your-aws-access-key>
export AWS_SECRET_ACCESS_KEY=<your-aws-secret-key>
Terraform Basics
In this section we will learn some basic concept and keywords of Terraform.
Provider
Terraform uses providers to interact with various cloud and infrastructure services. In our case it is AWS. We can define provider like this in our terraform file.
provider "aws" {
region = "us-east-1"
}
You can read about all providers here in this documentation.
Resources
Resources are the fundamental building blocks in Terraform. They represent the cloud infrastructure components you want to create or manage. To define resources in Terraform we can create block like this
resource "aws_instance" "server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
Here aws_instance is the resource name for creating EC2 and server is how we are going to refer this block in our file further. To know more about types of AWS resources check this documentation.
Variables
Variables in Terraform allow you to parameterize your configurations. They are placeholders for values that can change depending on the environment or use case. We can define variable like this.
variable "instance_type" {
description = "The type of EC2 instance to launch"
default = "t2.micro"
}
Then we can refer this variable in the ec2 field from var keyword like this var.instance_type. To further learn about types of variables follow this documentation
Outputs
Outputs in Terraform allow you to expose information about your infrastructure that you want to share or use in other configurations. Use the output keyword to declare outputs.
output "instance_ip" {
value = aws_instance.example.public_ip
}
To know in detail about output check this page
Terraform Lifecyle
Terraform's lifecycle refers to the stages that Terraform goes through when managing infrastructure. In short, Terraform's lifecycle consists of:
Initialize: This stage initializes the working directory and downloads the necessary provider plugins. It prepares Terraform for configuration.
terraform init
terraform plan
Apply: Applying the execution plan created during planning actually makes changes to your infrastructure. It creates, updates, or destroys resources as necessary to match the desired state.
terraform apply
terraform destroy
These lifecycle stages are essential for safely and efficiently managing infrastructure with Terraform, allowing you to plan changes, apply them, and maintain your desired infrastructure state.
Provisioning of EC2 instance
Now since we are aware of basics of terraform, We can automate the infrastructure process with it. For provisioning the infrastructure, In your terraform main.tf file use the code defined below. We are assuming you have created security key with name tf-webserver to ssh into instance.
provider "aws" {
region = "us-east-1"
}
# create a ec2 instance
resource "aws_instance" "webserver" {
ami = "ami-053b0d53c279acc90"
instance_type = "t2.micro"
security_groups = [aws_security_group.sg.name]
key_name = "tf-webserver"
tags = {
Name: "flask-app"
}
user_data = file("../scripts/env.sh")
}
# variable to use in security group for ingress
variable "ingress" {
type = list(number)
default = [80,443,22,3000]
description = "security group ports"
}
# variable to use in security group for egress
variable "egress" {
type = list(number)
default = [80,443]
description = "security group ports"
}
# Create security group resource
resource "aws_security_group" "sg" {
name = "Allow web traffic"
# create multiple ingress blocks with help of iterators
dynamic "ingress" {
iterator = port
for_each = var.ingress
content {
from_port = port.value
to_port = port.value
protocol = "TCP"
cidr_blocks = ["0.0.0.0/0"]
}
}
# create multiple egress blocks with help of iterators
dynamic "egress" {
iterator = port
for_each = var.egress
content {
from_port = port.value
to_port = port.value
protocol = "TCP"
cidr_blocks = ["0.0.0.0/0"]
}
}
}
#output public ip
output ec2-id {
value = aws_instance.webserver.public_ip
description = "ec2 instance public ip"
}
Check the outcome of script by running
terraform plan
If everything looks good run
terraform apply --auto-approve
To destroy the infrastructure run
terraform destroy
In this Blog we have learned what is Terraform, Basics of Terraform, Terraform lifecycle and how to provision AWS instance using Terraform. In our coming blogs we will discuss terraform state file and best practices to follow in terraform. Till then Have a great Learning
Peace.......